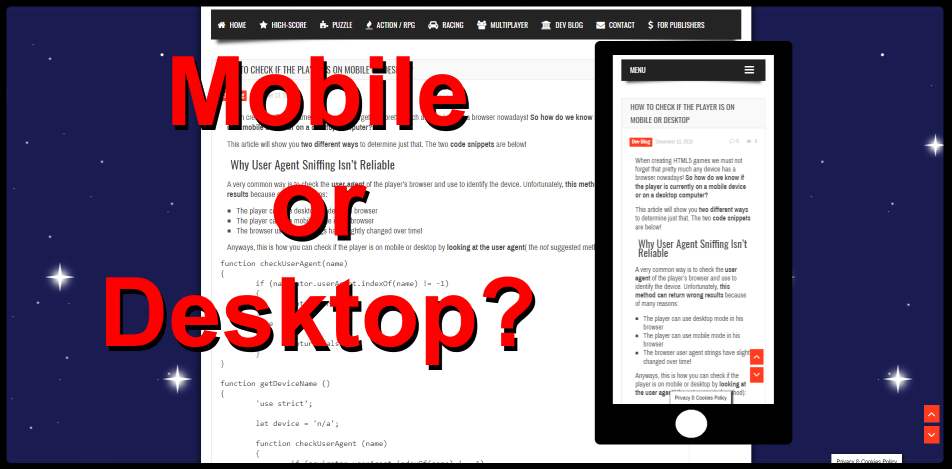
When creating HTML5 games we must not forget that pretty much any device has a browser nowadays! So how do we know if the player is currently on a mobile device or on a desktop computer?
This article will show you two different ways to determine just that. The two code snippets are below!
Why User Agent Sniffing Isn’t Reliable
A very common way is to check the user agent of the player’s browser and use to identify the device. Unfortunately, this method can return wrong results because of many reasons:
- The player can use desktop mode in his browser
- The player can use mobile mode in his browser
- The browser user agent strings have slightly changed over time!
Anyways, this is how you can check if the player is on mobile or desktop by looking at the user agent( the not suggested method):
function checkUserAgent(name) { if (navigator.userAgent.indexOf(name) != -1) { return true; } else { return false; } } function getDeviceName () { 'use strict'; let device = 'n/a'; function checkUserAgent (name) { if (navigator.userAgent.indexOf(name) != -1) { return true; } else { return false; } } if (checkUserAgent('Android')) { device = 'Android'; } else if (checkUserAgent('iPhone') && !window.MSStream) { device = 'iPhone'; } else if (checkUserAgent('Mac OS') || checkUserAgent('Macintosh')) { device = 'Mac OS'; } else if (checkUserAgent('Windows')) { device = 'Windows'; } return device; }
A Better Way to Check if it’s Mobile or Desktop
Now that we’ve seen the less ideal way of doing it, let’s look at an alternative approach that brings much better results.
First of all, I think it’s important to take a second and think about why we want to know if the player is on a mobile phone or on desktop. I would say that in most cases it is because of touch input!
So wouldn’t it be better to check for touch input? And if the device doesn’t have any touch input, then we don’t need to worry about it? Personally, I believe so, too!
Secondly, the good news is that we absolutely CAN check for touch input. And it is also much simpler!
Finally, this is the code I use in my index.html file to quickly determine if the player is using touch input (mobile devices) or not (desktop with keyboard & mouse):
<script> // Check touch input var IS_TOUCH = false; window.addEventListener('touchstart', function() { IS_TOUCH = true; }); </script>
Make sure that the very first screen your player see is a screen that says something along the lines of “Click to continue”. This way you can check for touch input at the earliest point and then handle it accordingly in the rest of the game.
And that’s all you need! You can check for <code>IS_TOUCH</code> in your game wherever you want to have different behaviors or controls for desktop & mobile.
The Ultimate Phaser 3 Guide
Everything you need to make real, polished, professional Phaser 3 games, you find it in this guide here: HTML5 Game Development Mini-Degree
I highly suggest you check it out!
What a great idea for checking it in what device!
Hey ReydVires, thanks for your comment! Glad you can use this method, it has always worked great for me, too 🙂